Im trying to import envirronement variables, im currently in a dilemma where I saw a section in the course at landgraf.dev which talks about the .ini file for Xdata but nothing on TMS Web Core.
What I want to know is, using the same method for both frameworks, what would be the best way: ini file or optset? I need basic parameters such as db credentials and url ip, ports and such.
Thanks
In WebCore or on the web, the local file system is not accessed. The data is on the "web". But of course an "ini" file can also be installed there.
This is how we do it:
(You can call this with a success proc as parameter)
unit Web.AppConfig;
interface
uses
JS,
{$IFDEF Miletus}
WEBLib.Miletus;
{$ELSE}
XData.Web.Connection,
XData.Web.Request,
XData.Web.Response;
{$ENDIF}
type
TAppConfig = class
private
FSystemScheme : string;
FSystemHost : string;
FRESTPort : Integer;
FBasisPath : string;
function GetBasisURL: string;
public
constructor Create;
procedure Assign( ASource: TAppConfig);
property BasisURL: string read GetBasisURL;
property SystemScheme : string read FSystemScheme;
property SystemHost : string read FSystemHost;
property RESTPort : Integer read FRESTPort;
property BasisPath : string read FBasisPath;
end;
TConfigLoadedProc = reference to procedure(Config: TAppConfig);
procedure WebAppLoadConfig( LoadProc: TConfigLoadedProc);
[async] function WebAppLoadConfigAsync: TAppConfig;
implementation
uses
System.SysUtils,
sng.ApiConsts,
sng.Consts,
sng.Settings;
{ TAppConfig }
constructor TAppConfig.Create;
begin
FSystemScheme := '--NoConfig-- https://';
FSystemHost := '--NoConfig-- localhost';
FRESTPort := 6040;
FBasisPath := 'Fehler /sopha';
end;
procedure TAppConfig.Assign( ASource: TAppConfig);
begin
FSystemScheme := ASource.SystemScheme;
FSystemHost := ASource.SystemHost;
FRESTPort := ASource.RESTPort;
FBasisPath := ASource.BasisPath;
end;
function TAppConfig.GetBasisURL: string;
begin
Result := FSystemScheme + FSystemHost + ':' + IntToStr(FRESTPort) + FBasisPath;
end;
{$IFDEF Miletus}
procedure WebAppLoadConfig( LoadProc: TConfigLoadedProc);
var
ini: TMiletusINIFile;
Config: TAppConfig;
begin
Config := TAppConfig.Create;
ini := TMiletusINIFile.Create( 'Path to ini xxx.ini');
try
Config.FSystemScheme := TAwait.ExecP<String>( ini.ReadString( cIniSecSystem, cIniSystemScheme, Config.FSystemScheme));
Config.FSystemHost := TAwait.ExecP<String>( ini.ReadString( cIniSecSystem, cIniSystemHost, Config.FSystemHost));
Config.FRESTPort := TAwait.ExecP<Integer>( ini.ReadInteger( cIniSecSystem, cIniRESTPort, Config.FRESTPort));
Config.FBasisPath := TAwait.ExecP<String>( ini.ReadString( cIniSecSystem, cIniBasisPath, Config.FBasisPath));
finally
LoadProc( Config);
ini.Free;
Config.Free;
end;
end;
{$ELSE}
procedure WebAppLoadConfig( LoadProc: TConfigLoadedProc);
procedure OnSuccess(Response: IHttpResponse);
var
Obj: TJSObject;
Config: TAppConfig;
begin
Config := TAppConfig.Create;
try
if Response.StatusCode = _http_200_Ok then begin
Obj := TJSJSON.parseObject( Response.ContentAsText);
if JS.toString( Obj[ cIniSystemScheme ]) <> '' then Config.FSystemScheme := JS.toString( Obj[ cIniSystemScheme ]);
if JS.toString( Obj[ cIniSystemHost ]) <> '' then Config.FSystemHost := JS.toString( Obj[ cIniSystemHost ]);
if JS.toInteger( Obj[ cIniRESTPort ]) <> 0 then Config.FRESTPort := JS.toInteger( Obj[ cIniRESTPort ]);
if JS.toString( Obj[ cIniBasisPath ]) <> '' then Config.FBasisPath := JS.toString( Obj[ cIniBasisPath ]);
end;
finally
LoadProc( Config);
Config.Free;
end;
end;
procedure OnError;
var
Config: TAppConfig;
begin
Config := TAppConfig.Create;
try
LoadProc( Config);
finally
Config.Free;
end;
end;
var
Conn: TXDataWebConnection;
ConfigPath: String;
begin
Conn := TXDataWebConnection.Create(nil);
try
Conn.SendRequest(THttpRequest.Create( cPathToConfig + cConfigFile), THttpResponseProc( @OnSuccess), THttpErrorProc( @OnError));
finally
Conn.Free;
end;
end;
{$ENDIF}
function WebAppLoadConfigAsync: TAppConfig;
var
Conn: TXDataWebConnection;
Response: IHttpResponse;
Obj: TJSObject;
begin
Result := nil;
Conn := TXDataWebConnection.Create(nil);
try
Response := TAwait.Exec<IHttpResponse>( Conn.SendRequestAsync( THttpRequest.Create( cPathToConfig + cConfigFile)));
if Response.StatusCode = _http_200_Ok then begin
Obj := TJSJSON.parseObject( Response.ContentAsText);
Result := TAppConfig.Create;
if JS.toString( Obj[ cIniSystemScheme ]) <> '' then Result.FSystemScheme := JS.toString( Obj[ cIniSystemScheme ]);
if JS.toString( Obj[ cIniSystemHost ]) <> '' then Result.FSystemHost := JS.toString( Obj[ cIniSystemHost ]);
if JS.toInteger( Obj[ cIniRESTPort ]) <> 0 then Result.FRESTPort := JS.toInteger( Obj[ cIniRESTPort ]);
if JS.toString( Obj[ cIniBasisPath ]) <> '' then Result.FBasisPath := JS.toString( Obj[ cIniBasisPath ]);
end;
finally
Conn.Free;
end;
end;
end.
But with this config are you able to switch easily the build config? Meaning can you switch rapidly from localhost to a distant server from here (see picture)
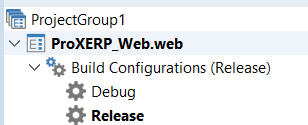
I have different config files
THttpRequest.Create( cPathToConfig + cConfigFile)
This can be simple txt-files